Text Manipulation Kung Fu for the Aspiring Black Belt
May 3rd, 2024
In the realm of software development, a text editor is not just a tool, but an extension of the programmer. Like martial artists who maneuver their swords with precision and grace, programmers wield their text editors, slicing through lines of text with deliberate intention. Consider this guide your dojo, a place where you'll hone your skills in the fine art of text manipulation.
We'll start with the basics of navigation and selection before embarking on a series of practical exercises. You'll be given some text, a goal for transforming that text, and guidance on where to find the necessary text manipulation commands for the task at hand. Solutions to the exercises will be provided in the form of screen capture videos, alongside detailed breakdowns of the commands used, allowing you to follow along and deepen your understanding.
Let's get into the basics of navigating and selecting.
Footwork: Cursor navigation and selection building blocks
The command palette is one of your best resources for discovering new commands and learning their associated keyboard shortcuts; open the command palette with cmd-shift-p
. The command palette allows for fuzzy searching, so you can type a few characters of a command you're looking for and quickly find it.
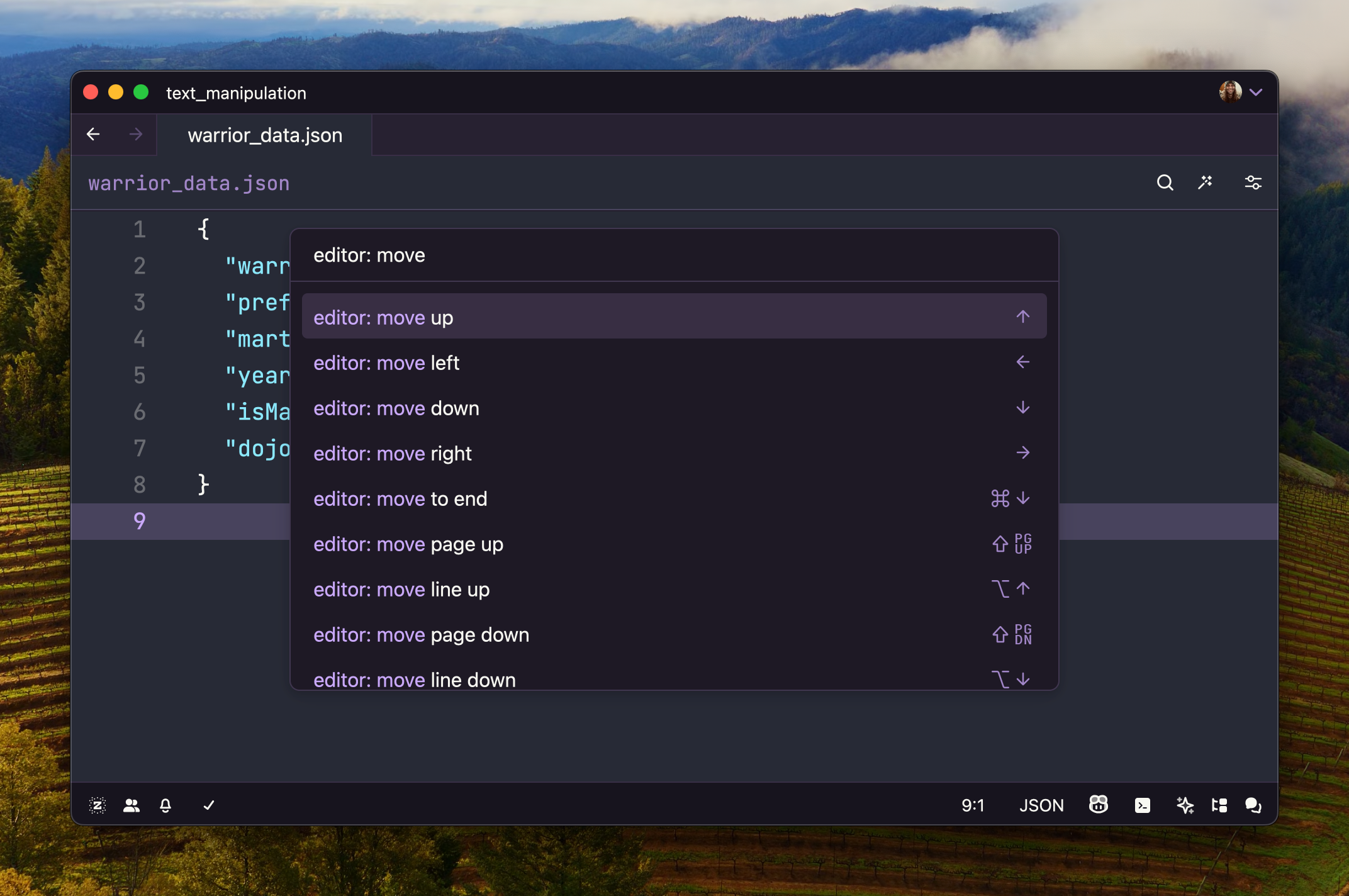
Within the command palette, if you search for editor: move
or editor: select
, you are going to find heaps of commands - commands for jumping to the beginning of a file, the end of a line, the start of a word, etc. You'll also see commands for selecting the next word, the next line, the next paragraph, etc. Learning all of these commands directly is both daunting and unnecessary. Instead, we'll focus on the learning the building blocks of cursor navigation and selection; these blocks can be intuitively combined to achieve more complex movements or selections.
Directional keys
left
: move cursor leftright
: move cursor rightup
: move cursor updown
: move cursor down
Modifiers
cmd
: used to specify jumping to some "edge" boundary in the editor. Ex:cmd-left
jumps to the beginning of the current line andcmd-up
jumps to the beginning of the file. Can you guess whatcmd-right
andcmd-down
do?alt
: used to specify jumping between text separated by "word boundaries." Word boundaries often occur when encountering non-alphanumeric characters, such as spaces or punctuation marks. Ex:alt-left
jumps to the beginning of the current word andalt-right
jumps to the end of it.alt
, when combined withctrl
, jumps between subwords, which is useful for jumping between the subwords of camelCase and snake_case variables.shift
: modifies the move to become a selection instead. Ex:shift-left
selects to the left andshift-right
selects to the right, rather than simply moving. Shift can be combined with other modifiers, likecmd-shift-left
to select to the beginning of the line.
Now that you've learned the building blocks of cursor navigation and selection commands, let's begin your training. When solving challengs, use a combination of digging into the command palette and intuition based on the aforementioned building blocks! Try your best to avoid using your mouse!
White Belt Challenge
Objective:
As you meticulously review your teammate's pull request, you notice a key in a JSON file that deviates from the naming convention used for the other keys. Your quest is to unify the keys to use the same naming convention!
Tips for your journey:
- Review cursor navigation and selection commands.
- Search for
convert case
in the command palette.
{
"warrior_name": "Takamura Hajime",
"preferred_weapon": "Yari",
"martial_art_form": "Jujitsu",
"years_of_training": 5,
"isMasterLevelCertified": false,
"dojo_affiliated_region": "Echigo"
}
Technique
Dissection
- Navigate to the beginning of
isMasterLevelCertified
. - Select
isMasterLevelCertified
viaeditor: select to next word end
. - Run
editor: convert to snake case
.
Honing your grip
Zed ships with some extremely useful text manipulation commands - many come in handy when translating between naming conventions of different programming lanugages:
editor: convert to lower camel case
editor: convert to upper camel case
editor: convert to lower case
editor: convert to upper case
editor: convert to kebab case
editor: convert to snake case
editor: convert to title case
editor: transpose
Green Belt Challenge
Objective:
You're working on an awesome Python program. You notice your train_fighter
function has a local variable, power_increase_factor
, that is read-only, and can be made into a global constant. Hoist the power_increase_factor
variable to the global scope and rename all instances of it to POWER_INCREASE_FACTOR
, as per the Python convention for global constants.
Tips for your journey:
- Review cursor navigation and selection commands.
- Search for
move line
in the command palette. - Search for
select all matches
in the command palette. - Search for
convert case
in the command palette.
def train_fighter(fighter_name, current_power):
power_increase_factor = 1.15
new_power = current_power * power_increase_factor
print(f"After arduous training, {fighter_name} has increased their power to {new_power:.2f}!")
return new_power
def display_fighter_stats(fighter_name, fighter_power):
print(f"{fighter_name}'s Current Power: {fighter_power}")
if __name__ == "__main__":
fighter_name = "Iron Fist"
initial_power = 100
display_fighter_stats(fighter_name, initial_power)
new_power_level = train_fighter(fighter_name, initial_power)
display_fighter_stats(fighter_name, new_power_level)
Technique
Dissection
- Add 2 newlines to the beginning of the file.
- Move cursor down to the beginning of the
power_increase_factor...
line. - Move the line up to the beginning of the file via
editor: move line up
. - Remove the indentation in the line via
editor: tab prev
. - Select
power_increase_factor
viaeditor: select to next word end
. - Select all instances of
power_increase_factor
viaeditor: select all matches
. - Run
editor: convert to upper snake case
.
Honing your grip
There are a many line manipulation commands available within Zed. When you have a list, sorting items and removing duplicates are common tasks that can be done with a few keystrokes:
editor: delete line
editor: duplicate line up
editor: duplicate line down
editor: join lines
editor: move line up
editor: move line down
editor: reverse lines
editor: shuffle lines
editor: sort lines case insensitive
editor: sort lines case sensitive
editor: unique lines case insensitive
editor: unique lines case sensitive
There are many ways to deploy multiple cursors to the editor in Zed; experiment with the following commands - all are useful in different scenarios:
editor: select all matches
editor: select next
editor: split selection into lines
editor: add selection above
editor: add selection below
editor: undo selection
- column-based selection -
alt-shift-mouse-drag
Brown Belt Challenge
Objective:
You've unearthed an ancient scroll (a CSV file) that lists information about historical martial arts masters. The CSV file has some inconsistencies that need to be corrected. You must clean the CSV file. Remove quotations and capitalize all the strings.
Tips for your journey:
- Review cursor navigation and selection commands.
- Review line manipulation commands.
- Review multi-cursor commands.
id,first_name,last_name,style,birth,death,country
1,"Miyamoto","Musashi","Swordsmanship",1584,1645,"japan"
2,yamamoto,tsunetomo,"Hagakure",1659,1719,"Japan"
3,"Ip","Man","wing Chun",1893,1972,"china"
4,morihei,ueshiba,Aikido,1883,1969,"Japan"
5,"Gichin","funakoshi","karate",1868,1957,"Japan"
Technique
Dissection
- Navigate to the first
"
. - Select the
"
viaeditor: select right
. - Select all instances of
"
viaeditor: select all matches
. - Delete the
"
s viaeditor: delete
. - Exit multi-cursor mode via
editor: cancel
. - Select the preceding
,
viaeditor: select left
. - Select all instances of
,
. We will not be usingeditor: select all matches
here, as we don't want to select the commas separating the column names. Instead, we will hold down the binding foreditor: select next
and repeatedly select the next match. Notice that if we select too many commas, we can undo selections viaeditor: undo selection
. - Dismiss the selections via
editor: move right
. - Select all of the words that directly proceed each commas via
editor: select to next word end
. Note that we will end up selecting some integers as well, but this won't matter in this case. - Convert the selected text to title case via
editor: convert to title case
.
Black Belt Challenge
Objective:
Extract the names of the martial art actors from the text below into a Markdown list, where each name is capitalized, put into <last>, <first>
format, and sorted alphabetically.
Tips for your journey:
- Review cursor navigation and selection commands.
- Review line manipulation commands.
- Review multi-cursor commands.
# Iconic Kung Fu Actors
## bruce lee
Bruce Lee's portrayal of "Lee" in the 1973 martial arts film *Enter the Dragon* is legendary. This character showcased Lee's exceptional martial arts skills, his philosophy of Jeet Kune Do, and his incredible physical prowess. The film cemented Bruce Lee's status as a global icon and greatly influenced the perception of Asian and martial arts culture in the Western world.
## jackie chan
Jackie Chan brought a new dimension to the martial arts film genre with his role as Wong Fei-hung in the 1978 film *Drunken Master*. Chan's unique blend of impressive martial arts and comedic timing created a refreshing and influential approach to the genre, highlighting the traditional drunken fist fighting style and establishing Chan as a major star in both Asia and the West.
## jet li
In the 1991 film *Once Upon a Time in China*, Jet Li portrays Huo Yuanjia, a revered martial arts master and founder of the Chin Woo Athletic Association. Li's performance was widely praised for its combination of grace, agility, and martial arts expertise, offering a narrative that explored Chinese nationalism and the importance of martial arts in cultural identity.
## donnie yen
Donnie Yen's role as Ip Man, the Wing Chun grandmaster, and teacher of Bruce Lee, in the 2008 film *Ip Man*, is considered one of his best performances. Through this role, Yen was able to showcase the skill, philosophy, and humanity of Ip Man, portraying significant events in his life against the backdrop of the Sino-Japanese War. The film series has become a classic, highlighting the spirit of Wing Chun and its significance in martial arts history.
## michelle yeoh
Michelle Yeoh's portrayal of Yu Shu Lien in the 2000 film *Crouching Tiger, Hidden Dragon* brought her international acclaim. Her character is a skilled warrior who navigates a complex plot of love, honor, and betrayal. Yeoh's performance, alongside her martial arts skill, played a significant role in the film's success, demonstrating the power and depth of female characters in the martial arts genre.
Technique
Dissection
- Navigate down to the first
##
header. - Select
##
viaeditor: select right
. - Select all instances of
##
viaeditor: select all matches
. - Dismiss the selections via
editor: move right
. - Select to the end of each line via
editor: select to end of line
. - Copy each selection via
editor: copy
. - Navigate to the end of the document via
editor: move to end
. - Insert a few newlines via
editor: newline
. - Paste the copied text via
editor: paste
. - Select the final line via
editor: select to beginning of line
. - Expand the selection upward to the first name via multiptle
editor: select up
. - Run
editor: split selection into lines
to add a cursor to the end of each line. - Jump to the space in between each first and last name via
editor: move to previous word start
. - Delete the spaces via
editor: delete
. - Select all of the last names via
editor: select to next word end
. - Copy the last names via
editor: copy
. - Delete the last names via
editor: delete
. - Jump back to the beginning of each line via
editor: move to beginning of line
. - Paste the last names via
editor: paste
. - Add a
,
after each first name. - Jump to the beginning of each line via
editor: move to beginning of line
. - Select to the end of each line via
editor: select to end of line
. - Convert the selected text to title case via
editor: convert to title case
. - Jump back to the beginning of each line via
editor: move to beginning of line
. - Insert
- [ ]
before each name. - Jump to the end of each line via
editor: move to end
. - Select each line via
editor: select to beginning of line
. - Sort the lines via
editor: sort lines case sensitive
.
Conclusion
Transforming text can be as entertaining as it is useful. The process can be "gamified" by seeking solutions that require the fewest possible steps (you may have discovered more straightforward solutions for the exercises than those suggested). However, not all transformations are as clear cut as in the exercises. For example, it's often that some repetitive editing task can't be fully addressed in one fell swoop with multiple cursors, leaving a bit of manual work to be performed after. In these instances, the goal becomes finding the most efficient state for the text that minimizes manual follow-up.
We hope that you were able to pick up some new tricks and techniques to add to your text manipulation arsenal. Remember, the more you practice, the more proficient you'll become. So, keep honing your grip! Feel free to share with us any interesting manipulations you've deployed!